Reverse a Linked List in Python
Linked lists are one of the fundamental records systems in Computer science, and knowledge how to manipulate them is important for any aspiring developer. Among the various operations you could perform on a related list, reversing the list is a common and crucial mission. In this guide, we’ll discover what a connected list is, why reversing a related list is beneficial, and how you could reverse a linked list in Python the usage of extraordinary strategies. We’ll additionally stroll through every technique with code examples and explanations.
Table of Contents
What is a Linked List?
A linked list is a linear data structure where each element, known as a node, contains two components:
- Data: The value stored in the node.
- Next: A reference (or pointer) to the next node in the sequence.
Unlike arrays, linked lists do not store elements in contiguous memory locations. Instead, the nodes are scattered throughout memory and are connected by pointers. This structure allows for efficient insertion and deletion of elements, particularly when dealing with dynamic data sizes.
There are different types of linked lists, such as:
• Singly Linked List: Each node points to the next node, and the last node points to None.
• Doubly Linked List: Each node points to each the subsequent and the previous node.
• Circular Linked List: The closing node points again to the primary node, forming a circle.
For the purpose of this manual, we are able to attention on reversing a singly related list.
Why Reverse a Linked List?
- Reversing a linked list is a common hassle in technical interviews and coding challenges. The operation is beneficial in numerous situations, together with:
- Reversing the order of elements to meet specific criteria.
- Implementing certain algorithms that require list traversal in reverse order.
- Simplifying other operations by first reversing the list.
Now that we have a basic understanding of linked lists, let’s dive into how to reverse one.
Method 1: Iterative Approach
The iterative method is a straightforward and efficient way to reverse a linked list. It involves traversing the list and rearranging the pointers as we go.
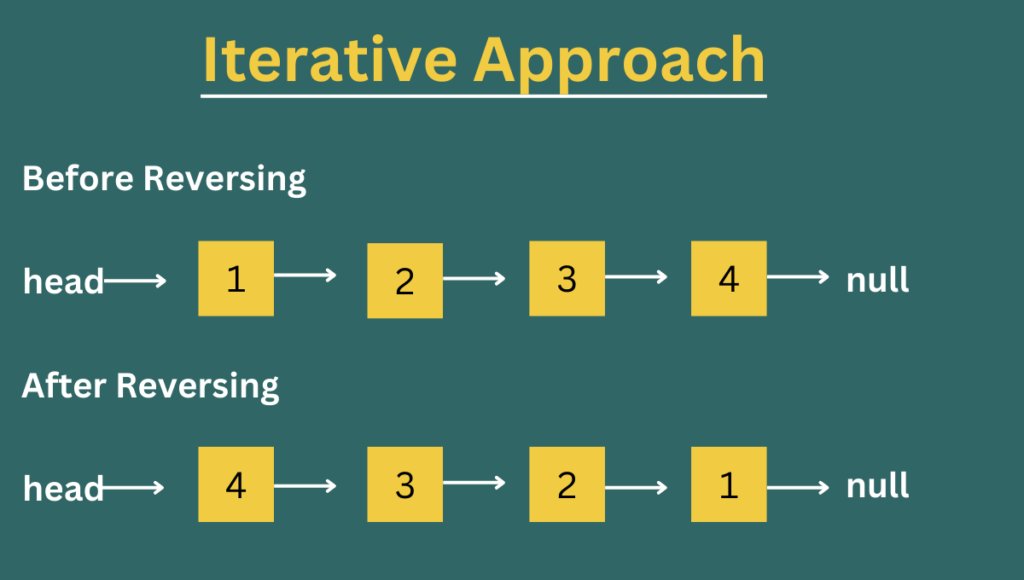
Step-by-Step Explanation
- Initialize Variables: Start by creating three pointers:
- prev (initially set to None)
- current (initially set to the head of the list)
- next (used to temporarily store the next node)
- Traverse the List: Loop through the linked list until the current node becomes None. For each node:
- Save the next node using next = current.next.
- Reverse the pointer of the current node by setting current.next = prev.
- Move the prev and current pointers one step forward.
- Update the Head: Once the loop is complete, set the head of the list to the prev pointer, which will be pointing to the new head of the reversed list.
Example Code
class Node:
def __init__(self, data):
self.data = data
self.next = None
class LinkedList:
def __init__(self):
self.head = None
def append(self, data):
new_node = Node(data)
if not self.head:
self.head = new_node
return
last = self.head
while last.next:
last = last.next
last.next = new_node
def reverse(self):
prev = None
current = self.head
while current:
next_node = current.next
current.next = prev
prev = current
current = next_node
self.head = prev
def print_list(self):
current = self.head
while current:
print(current.data, end=" -> ")
current = current.next
print("None")
# Example usage
ll = LinkedList()
ll.append(1)
ll.append(2)
ll.append(3)
ll.append(4)
print("Original Linked List:")
ll.print_list()
ll.reverse()
print("Reversed Linked List:")
ll.print_list()
Output
Original Linked List:
1 -> 2 -> 3 -> 4 -> None
Reversed Linked List:
4 -> 3 -> 2 -> 1 -> None
Explanation
- append method: Adds a new node to the end of the linked list.
- reverse method: Reverses the linked list using the iterative approach.
- print_list method: Prints the linked list in a readable format.
The iterative approach is efficient with a time complexity of O(n) and a space complexity of O(1), where n is the number of nodes in the list.
Method 2: Recursive Approach
The recursive method is an elegant and concise way to reverse a linked list. It involves reversing the rest of the list recursively and then fixing the pointers.
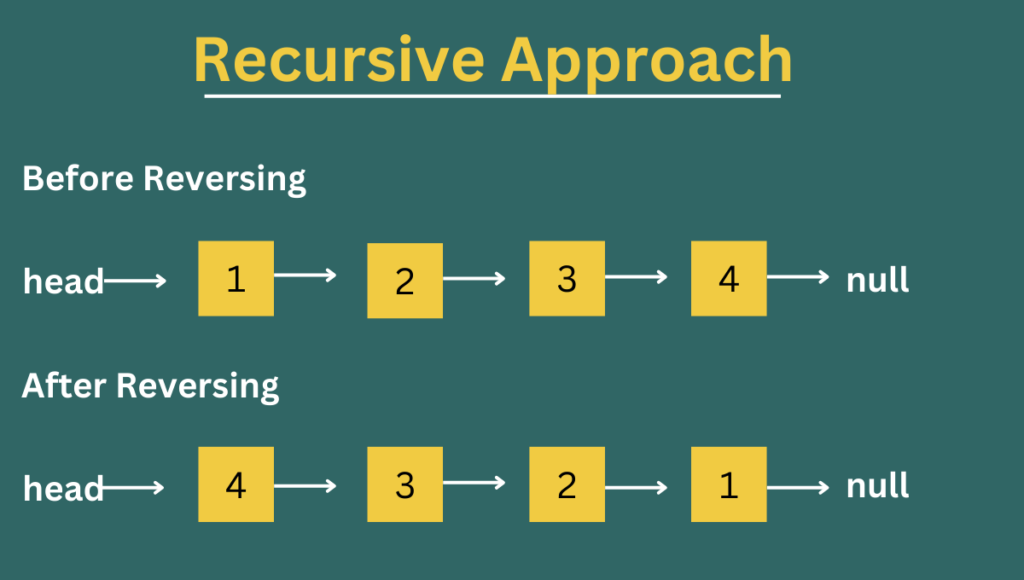
Step-by-Step Explanation
- Base Case: If the head is None or the next node is None, return the head. This indicates that the list is either empty or has reached the last node.
- Recursive Call: Recursively reverse the rest of the list.
- Fix Pointers: Once the recursion returns, the next node’s pointer should be reversed to point back to the current node.
- Set next to None: To avoid a circular reference, set the next pointer of the current node to None.
Example Code
class LinkedListRecursive:
def __init__(self):
self.head = None
def append(self, data):
new_node = Node(data)
if not self.head:
self.head = new_node
return
last = self.head
while last.next:
last = last.next
last.next = new_node
def reverse_recursive(self, node):
if not node or not node.next:
return node
new_head = self.reverse_recursive(node.next)
node.next.next = node
node.next = None
return new_head
def reverse(self):
self.head = self.reverse_recursive(self.head)
def print_list(self):
current = self.head
while current:
print(current.data, end=" -> ")
current = current.next
print("None")
# Example usage
ll = LinkedListRecursive()
ll.append(1)
ll.append(2)
ll.append(3)
ll.append(4)
print("Original Linked List:")
ll.print_list()
ll.reverse()
print("Reversed Linked List:")
ll.print_list()
Output
Original Linked List:
1 -> 2 -> 3 -> 4 -> None
Reversed Linked List:
4 -> 3 -> 2 -> 1 -> None
Explanation
- reverse_recursive method: Recursively reverses the linked list by flipping the pointers as the recursion unwinds.
- reverse method: Acts as a wrapper to initiate the recursive reversal.
The recursive approach also has a time complexity of O(n) but comes with a space complexity of O(n) due to the recursion stack.
Method 3: Using Stack (Alternative Approach)
Another way to reverse a linked list is by using a stack. This method is less common but can be useful in certain scenarios.
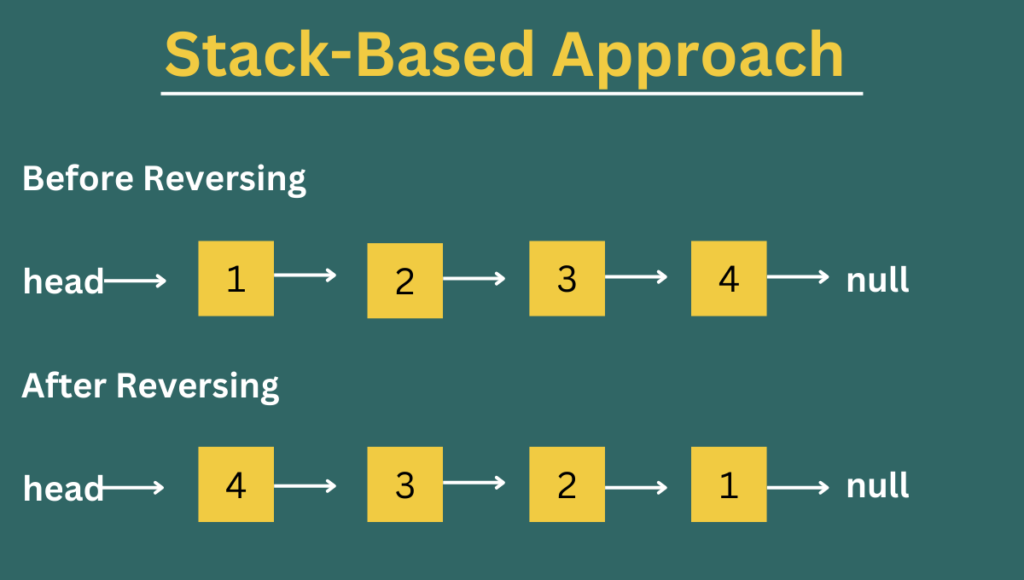
Step-by-Step Explanation
- Push Nodes onto the Stack: Traverse the linked list and push each node onto a stack.
- Pop Nodes from the Stack: Pop nodes from the stack one by one and rearrange the pointers to form the reversed list.
Example Code
class LinkedListStack:
def __init__(self):
self.head = None
def append(self, data):
new_node = Node(data)
if not self.head:
self.head = new_node
return
last = self.head
while last.next:
last = last.next
last.next = new_node
def reverse(self):
stack = []
current = self.head
while current:
stack.append(current)
current = current.next
self.head = stack.pop()
current = self.head
while stack:
current.next = stack.pop()
current = current.next
current.next = None
def print_list(self):
current = self.head
while current:
print(current.data, end=" -> ")
current = current.next
print("None")
# Example usage
ll = LinkedListStack()
ll.append(1)
ll.append(2)
ll.append(3)
ll.append(4)
print("Original Linked List:")
ll.print_list()
ll.reverse()
print("Reversed Linked List:")
ll.print_list()
Output
Original Linked List:
1 -> 2 -> 3 -> 4 -> None
Reversed Linked List:
4 -> 3 -> 2 -> 1 -> None
Explanation
• Stack-Based Reversal: This method leverages the Last In, First Out (LIFO) nature of a stack to opposite the related listing. Nodes are pushed onto the stack for the duration of traversal and then popped off in opposite order.
The stack-primarily based technique has a time complexity of O(n) and a area complexity of O(n) due to the stack garage.
Conclusion
Reversing a related listing is a essential operation that can be implemented the use of numerous methods, every with its own blessings. The iterative method is green and easy to recognize, whilst the recursive method offers a more elegant answer. The stack-primarily based method, though much less commonplace, may be a beneficial opportunity in particular cases.
FAQS
What is a linked list in Python?
A linked list is a data structure where each element (called a node) contains a reference (or link) to the next node in the sequence. Unlike arrays, linked lists do not store elements in contiguous memory locations.
Why would I need to reverse a linked list?
Reversing a linked list is a common operation that may be necessary in various algorithms. For example, reversing a linked list could be useful in scenarios where you need to traverse a list in reverse order without using extra space.
What are the different methods to reverse a linked list in Python?
There are several methods to reverse a linked list in Python, including:Iterative Approach: Using a loop to reverse the direction of the links.
Recursive Approach: Using the call stack to reverse the list.
Stack-Based Approach: Using an external stack to reverse the elements.
Which approach is the most efficient for reversing a linked list?
The iterative approach is generally the most efficient in terms of time and space complexity. It operates in O(n) time and uses O(1) additional space. The recursive approach also has a time complexity of O(n) but uses O(n) space due to the call stack.
NOTE: You can also read
Как избежать дубликатов номеров в документах, как поступить?
Чем опасны дубликаты номеров в базе данных, подскажите.
Как проверить документ на наличие дубликатов номеров, дайте рекомендации.
Эффективные методы очистки системы от повторений номеров, подскажите.
Эффективные способы избежать одинаковых номеров в таблице, подскажите.
Как избежать дублирования номеров при составлении отчета, подскажите.
Эффективные шаги по устранению повторяющихся номеров в системе, расскажите.
Как избежать повторений номеров при создании текстов, расскажите.
Как избавиться от одинаковых номеров, подскажите.
Как избежать повторений номеров при написании документов, расскажите.
изготовление номера на автомобиль http://www.avtonomera77.su/ .