C++ Arrays
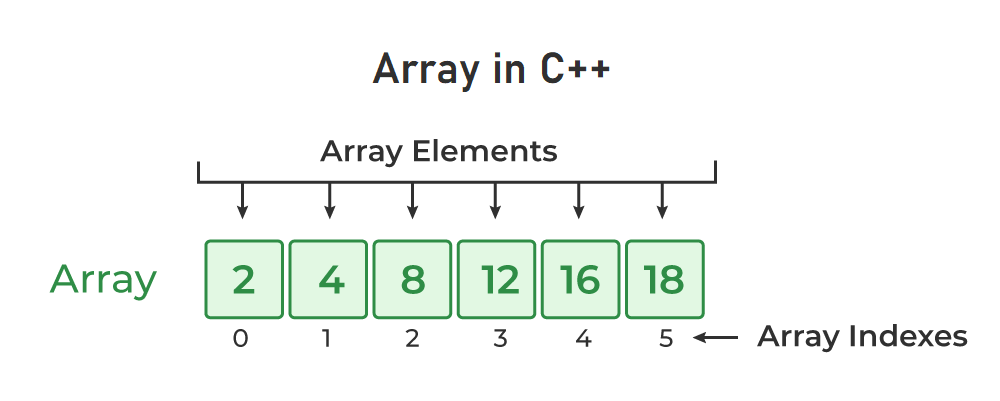
Table of Contents
In C++ Array in a data structure that allows you to store a fixed-size sequence of elements of the same type in touching memory locations. Rows provide a (producing a lot with very little waste) way to manage collections of related data, enabling quick access and moving around/misleading and tricking of individual elements through their index. For instance, you might use an organized row to store a list of student grades or a series of temperature readings.
Declaring an organized row involves specifying the type of its elements, the organized row name, and its size. Once declared, you can initialize the organized row with clearly stated/particular values and perform different operations such as insertion, deletion, searching, and sorting. Rows are a basic idea in C++ programming, forming the basis for more advanced data structures and sets of computer instructions.
C++ Arrays are basic in C++ programming, providing a way to store and manage collections of data. Whether you’re working on simple tasks or complex sets of computer instructions, rows offer a structured approach to handling data (in a way that produces a lot with very little waste). In this article, we’ll dive deep into the world of C++ rows, exploring their (official, public statement/document with such a statement), initialization, and different operations with practical examples.
1. Introduction to Arrays
An organized Array is a collection of elements, all of the same type, stored in touching memory locations. Rows allow you to store many values in a single (number or thing that changes), making it easier to manage and control/move around/mislead related data. For instance, if you need to store the grades of 30 students, an organized row can be a convenient solution.
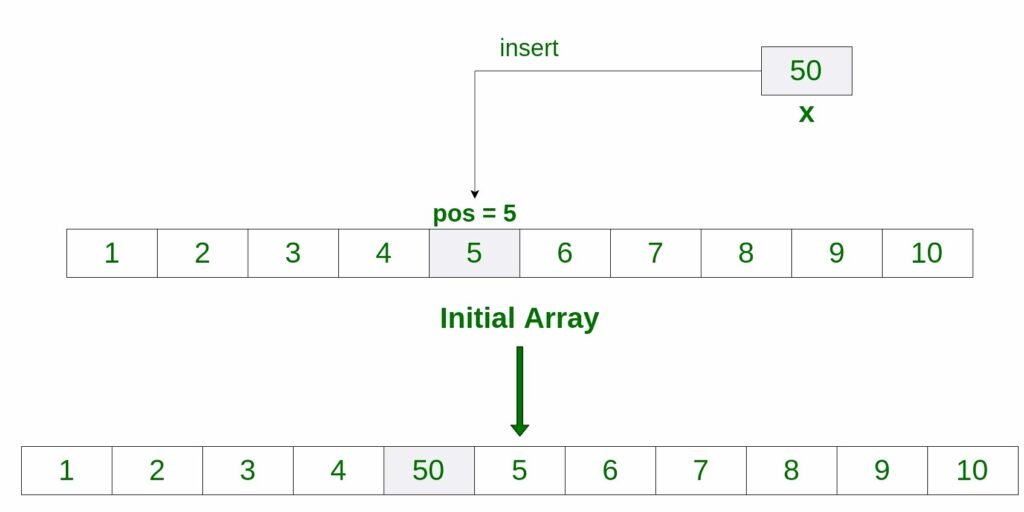
2. Declaring and Initializing Arrays
Declaring Arrays
In C++, you declare an array by specifying the type of its elements, the array name, and the number of elements it will hold. Here’s the basic syntax:
type arrayName[arraySize];
For example, to declare an array of integers with 5 elements:
int grades[5];
Initializing Arrays
You can initialize an array at the time of declaration:
int grades[5] = {85, 90, 78, 92, 88};
If you omit the size, the compiler will determine it based on the number of elements:
int grades[] = {85, 90, 78, 92, 88};
3. Accessing Array Elements
Array elements are accessed using their index, starting from 0. For example, to access the first element of the grades array:
int firstGrade = grades[0];
You can also modify an element by specifying its index:
grades[2] = 80; // Changes the third element to 80
Here’s a complete example that demonstrates array declaration, initialization, and element access:
#include <iostream>
using namespace std;
int main() {
int grades[5] = {85, 90, 78, 92, 88};
// Accessing and modifying elements
cout << "First grade: " << grades[0] << endl;
grades[2] = 80;
cout << "Updated third grade: " << grades[2] << endl;
return 0;
}
4. Common Array Operations
Insertion
Inserting elements into a clearly stated/particular position in an organized row can be tricky because rows have a fixed size. You need to shift elements to make room for the new element. Here’s an example of inserting an element into an organized row:
#include <iostream>
using namespace std;
void insertElement(int arr[], int &n, int pos, int element) {
for (int i = n; i > pos; --i) {
arr[i] = arr[i - 1];
}
arr[pos] = element;
n++;
}
int main() {
int grades[6] = {85, 90, 78, 92, 88};
int n = 5;
int pos = 2;
int newGrade = 80;
insertElement(grades, n, pos, newGrade);
cout << "Array after insertion: ";
for (int i = 0; i < n; ++i) {
cout << grades[i] << " ";
}
cout << endl;
return 0;
}
Deletion
Deleting an element involves shifting the elements after it to fill the gap. Here’s an example:
#include <iostream>
using namespace std;
void deleteElement(int arr[], int &n, int pos) {
for (int i = pos; i < n - 1; ++i) {
arr[i] = arr[i + 1];
}
n--;
}
int main() {
int grades[5] = {85, 90, 78, 92, 88};
int n = 5;
int pos = 2;
deleteElement(grades, n, pos);
cout << "Array after deletion: ";
for (int i = 0; i < n; ++i) {
cout << grades[i] << " ";
}
cout << endl;
return 0;
}
Searching
Searching for an element can be done using linear search. Here’s an example:
#include <iostream>
using namespace std;
int linearSearch(int arr[], int n, int element) {
for (int i = 0; i < n; ++i) {
if (arr[i] == element) {
return i;
}
}
return -1;
}
int main() {
int grades[5] = {85, 90, 78, 92, 88};
int n = 5;
int searchElement = 92;
int pos = linearSearch(grades, n, searchElement);
if (pos != -1) {
cout << "Element found at position: " << pos << endl;
} else {
cout << "Element not found." << endl;
}
return 0;
}
Sorting
Sorting an array can be achieved using various algorithms. Here’s an example using bubble sort:
#include <iostream>
using namespace std;
void bubbleSort(int arr[], int n) {
for (int i = 0; i < n - 1; ++i) {
for (int j = 0; j < n - i - 1; ++j) {
if (arr[j] > arr[j + 1]) {
swap(arr[j], arr[j + 1]);
}
}
}
}
int main() {
int grades[5] = {85, 90, 78, 92, 88};
int n = 5;
bubbleSort(grades, n);
cout << "Array after sorting: ";
for (int i = 0; i < n; ++i) {
cout << grades[i] << " ";
}
cout << endl;
return 0;
}
5. Dynamic Arrays
Static C++ Arrays have a fixed size, but sometimes you need more flexibility. Pattern of behavior rows allow you to set apart and distribute memory at runtime, which can grow or shrink as needed
Using new and delete
You can create dynamic arrays using the new operator:
#include <iostream>
using namespace std;
int main() {
int n;
cout << "Enter the size of the array: ";
cin >> n;
int* grades = new int[n];
for (int i = 0; i < n; ++i) {
cout << "Enter grade " << i + 1 << ": ";
cin >> grades[i];
}
cout << "Entered grades: ";
for (int i = 0; i < n; ++i) {
cout << grades[i] << " ";
}
cout << endl;
delete[] grades;
return 0;
}
In this example, we allocate memory for an array of size n at runtime and deallocate it using delete[].
Using std::vector
The C++ Standard Library provides the std::vector class, which offers dynamic array capabilities with additional functionalities. Here’s an example:
#include <iostream>
#include <vector>
using namespace std;
int main() {
vector<int> grades;
int n, grade;
cout << "Enter the number of grades: ";
cin >> n;
for (int i = 0; i < n; ++i) {
cout << "Enter grade " << i + 1 << ": ";
cin >> grade;
grades.push_back(grade);
}
cout << "Entered grades: ";
for (int i = 0; i < grades.size(); ++i) {
cout << grades[i] << " ";
}
cout << endl;
return 0;
}
std::vector is part of the C++ Standard Library and automatically manages memory, resizing as needed.
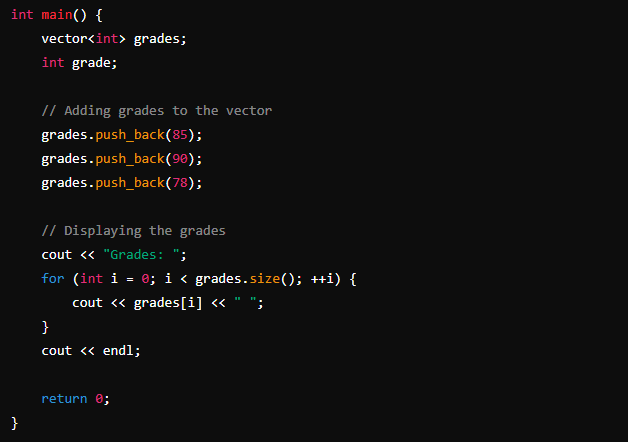
NOTE: You can alslo read Merge Sort Algorithm and Its Implementations
Conclusion
Arrays are a basic data structure in C++ Arrays, providing a way to store and manage collections of elements (in a way that produces a lot with very little waste). Understanding how to declare, initialize, and control/move around/mislead rows is extremely important for effective programming. We’ve covered the basics of rows, including common operations like insertion, deletion, searching, and sorting, along with energetic/changing rows using new and vector. With these tools, you’ll be well-prepared to handle a variety of data management tasks in your C++ programs.
FAQS
What is an array in C++?
An C++ Arrays is a data structure that allows you to store a fixed-size sequence of elements of the same type in contiguous memory locations. It provides an efficient way to manage and manipulate collections of related data.
How do you declare an array in C++?
To declare an array in C++, specify the type of its elements, the array name, and the number of elements it will hold.
How do you access elements of an array in C++?
Array elements are accessed using their index, starting from 0. For example, to access the first element of the grades
array.
6 thoughts on “C++ Arrays: Understanding and Implementing Arrays with Practical Examples”