How to Compare Two Arrays in JavaScript (Complete method)
Table of Contents
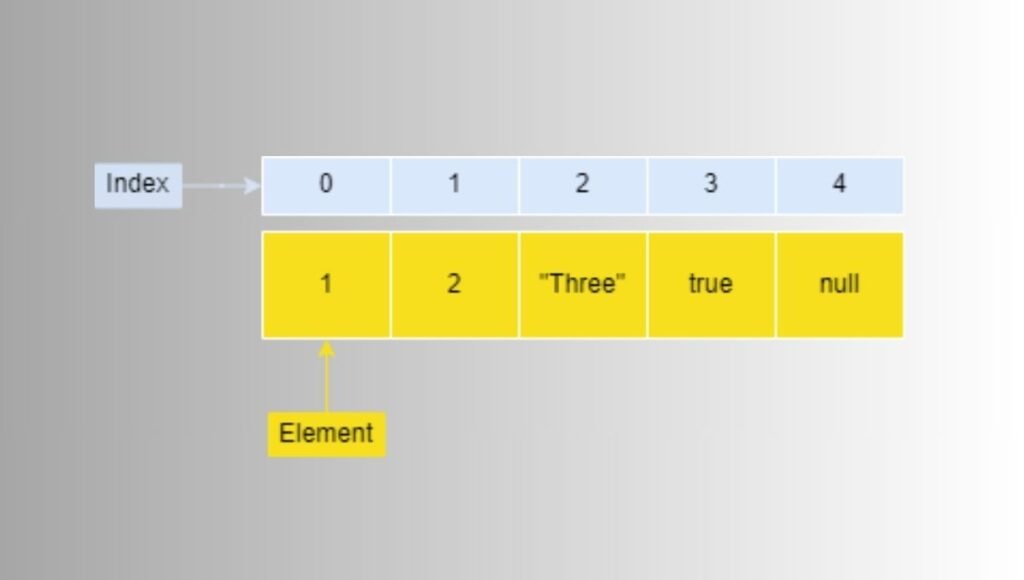
At first sight, comparing arrays in JavaScript looks pretty simple, but it becomes nuanced the further it goes. Be it for a personal project or something you are making for your client, you will definitely save yourself from some hours of time and headache if you know how to compare arrays. We’ll walk through various methods to compare two arrays in JavaScript in this guide—making sure you have the tools to do so for this common task.
Understanding the Basics
Before diving into the different ways to compare arrays, let’s define what “comparing arrays” even means. There are many different types of comparisons that one might want to do:
• Equality: Whether two arrays have the same elements in the same order.
• Shallow Equality: This will check whether two arrays have the same elements in them, order irrelevant.
• Deep Equality: Whether two arrays have the same elements in the same order. This includes nested arrays.
Each of these comparisons has cases of application and difficulty. Let’s look at how each type is implemented in JavaScript.
Equality: Checking if Two Arrays Are Identical
To check if two arrays are identical (i.e., they have the same elements in the same order), you can use the following approach:
function arraysAreEqual(arr1, arr2) {
if (arr1.length !== arr2.length) {
return false;
}
for (let i = 0; i < arr1.length; i++) {
if (arr1[i] !== arr2[i]) {
return false;
}
}
return true;
}
// Example Usage
const array1 = [1, 2, 3];
const array2 = [1, 2, 3];
console.log(arraysAreEqual(array1, array2)); // Output: true
const array3 = [1, 2, 3];
const array4 = [3, 2, 1];
console.log(arraysAreEqual(array3, array4)); // Output: false
This function first checks whether the lengths of the two arrays are equal. If they aren’t, then it definitely can’t be identical. It cycles through each element and checks them. If any discrepancy is found, then we return false. If we complete the loop without finding any differences, then we return true.
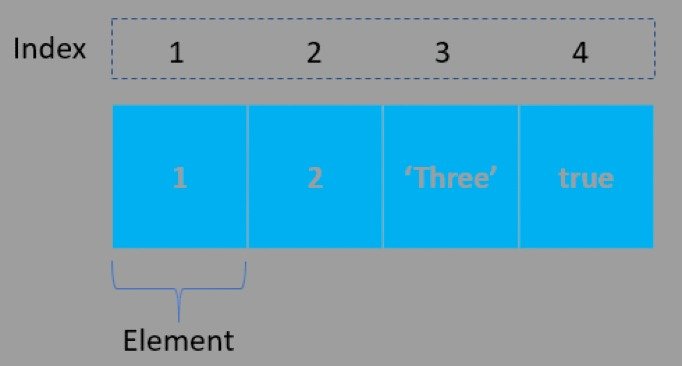
Shallow Equality: Ignoring Order
To check if two arrays have the same elements regardless of order, we can use sorting:
function arraysHaveSameElements(arr1, arr2) {
if (arr1.length !== arr2.length) {
return false;
}
const sortedArr1 = arr1.slice().sort();
const sortedArr2 = arr2.slice().sort();
for (let i = 0; i < sortedArr1.length; i++) {
if (sortedArr1[i] !== sortedArr2[i]) {
return false;
}
}
return true;
}
// Example Usage
const array5 = [1, 2, 3];
const array6 = [3, 2, 1];
console.log(arraysHaveSameElements(array5, array6)); // Output: true
const array7 = [1, 2, 3];
const array8 = [4, 5, 6];
console.log(arraysHaveSameElements(array7, array8)); // Output: false
In this approach, we will first sort both arrays and then compare them element by element. The slice () function is applied to avoid the mutation of the original arrays..
Deep Equality: Comparing Nested Arrays
For deep equality, when you need to take nested arrays or objects into consideration, you will need a more robust solution. Here is one using recursion:
function deepEqual(obj1, obj2) {
if (obj1 === obj2) {
return true;
}
if (obj1 == null || typeof obj1 !== "object" || obj2 == null || typeof obj2 !== "object") {
return false;
}
let keys1 = Object.keys(obj1);
let keys2 = Object.keys(obj2);
if (keys1.length !== keys2.length) {
return false;
}
for (let key of keys1) {
if (!keys2.includes(key) || !deepEqual(obj1[key], obj2[key])) {
return false;
}
}
return true;
}
function deepArrayEquality(arr1, arr2) {
if (arr1.length !== arr2.length) {
return false;
}
for (let i = 0; i < arr1.length; i++) {
if (!deepEqual(arr1[i], arr2[i])) {
return false;
}
}
return true;
}
// Example Usage
const nestedArray1 = [1, [2, 3], [4, [5, 6]]];
const nestedArray2 = [1, [2, 3], [4, [5, 6]]];
console.log(deepArrayEquality(nestedArray1, nestedArray2)); // Output: true
const nestedArray3 = [1, [2, 3], [4, [5, 7]]];
const nestedArray4 = [1, [2, 3], [4, [5, 6]]];
console.log(deepArrayEquality(nestedArray3, nestedArray4)); // Output: false
The deepEqual function compares two objects or arrays recursively. The deepArrayEquality function uses deepEqual to compare each element in the arrays.
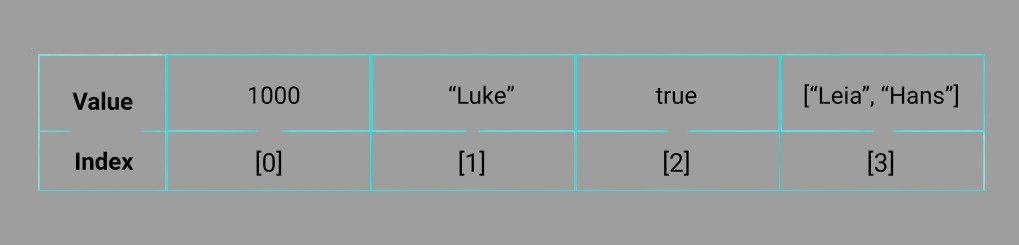
Performance Considerations
This can create performance issues when comparing arrays, particularly big ones. For instance, the time complexity of arranging an array is O(n log n), which is genuinely slow for very big arrays. Consider the size of the data being processed and the performance implication of the methods that are applied on it.
Using Array Methods and ES6 Features
Clearly, JavaScript array methods and ES6 features can make your life much easier. You might, for instance, use the every method for an even shorter equality check:
function arraysAreEqualES6(arr1, arr2) {
return arr1.length === arr2.length && arr1.every((value, index) => value === arr2[index]);
}
// Example Usage
const array9 = [1, 2, 3];
const array10 = [1, 2, 3];
console.log(arraysAreEqualES6(array9, array10)); // Output: true
const array11 = [1, 2, 3];
const array12 = [3, 2, 1];
console.log(arraysAreEqualES6(array11, array12)); // Output: false
The every method tests whether all elements in the array pass the provided function, making it a clean and readable solution for equality checks.
NOTE: you can also read
- Types of Image Segmentation Explained
- How a Motherboard Works : The Heart of Your Computer
- Mesh Topology: Complete Guide with Examples
- Star Topology: A Guide to Efficient Network Design
- What is MongoDB Compass
Conclusion
It can also be done in different ways in JavaScript, depending on the type of comparison that is to be made. The methods range from a simple equality check to a deep comparison between the nested arrays, each having its own cases and complexities. It is only with knowledge of the methods that you will make the right choices for your needs, hence assuring efficiency and effectiveness in your code.
At first sight, comparing arrays in JavaScript looks pretty simple, but it becomes nuanced the further it goes. Be it for a personal project or something you are making for your client, you will definitely save yourself from some hours of time and headache if you know how to compare arrays. We’ll walk through various methods to compare two arrays in JavaScript in this guide—making sure you have the tools to do so for this common task.
FAQS
What is the simplest way to compare two arrays for equality in JavaScript?
The simplest way to compare two arrays for equality, meaning they have the same elements in the same order, is by using a loop:
function arraysAreEqual(arr1, arr2) {
if (arr1.length !== arr2.length) return false;
for (let i = 0; i < arr1.length; i++) {
if (arr1[i] !== arr2[i]) return false;
}
return true;
}
How can I check if two arrays contain the same elements, regardless of order?
To check if two arrays contain the same elements regardless of order, you can sort the arrays first and then compare them:
function arraysHaveSameElements(arr1, arr2) {
if (arr1.length !== arr2.length) return false;
const sortedArr1 = arr1.slice().sort();
const sortedArr2 = arr2.slice().sort();
return sortedArr1.every((value, index) => value === sortedArr2[index]);
}
What is deep equality, and how do I compare nested arrays?
Deep equality means checking if two arrays contain the same elements in the same order, including nested arrays or objects. Here’s a function for deep array comparison:
function deepEqual(obj1, obj2) {
if (obj1 === obj2) return true;
if (obj1 == null || typeof obj1 !== “object” || obj2 == null || typeof obj2 !== “object”) return false;
let keys1 = Object.keys(obj1);
let keys2 = Object.keys(obj2);
if (keys1.length !== keys2.length) return false;
for (let key of keys1) {
if (!keys2.includes(key) || !deepEqual(obj1[key], obj2[key])) return false;
}
return true;
}
function deepArrayEquality(arr1, arr2) {
if (arr1.length !== arr2.length) return false;
for (let i = 0; i < arr1.length; i++) {
if (!deepEqual(arr1[i], arr2[i])) return false;
}
return true;
}
2 thoughts on “How to Compare Two Arrays in JavaScript (Complete method)”