7 AI Tools for Developers: Boosting Productivity and Innovation
Table of Contents
Artificial Intelligence (AI) has revolutionized various fields, and software development is no exception. The rise of AI tools for developers has significantly streamlined coding, debugging, and project management processes, enabling developers to build robust and innovative applications more efficiently. This article delves into some of the top AI tools that every developer should know, providing insights into their functionalities, use cases, and even some code snippets to get you started.
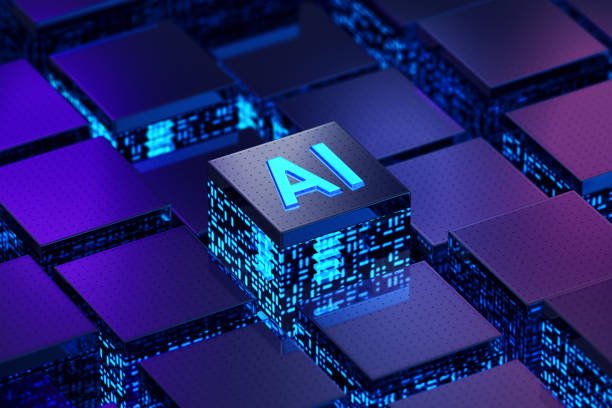
1. GitHub Copilot
GitHub Copilot, developed by GitHub and OpenAI, is an AI-powered code completion tool. It leverages the power of OpenAI’s Codex model to provide intelligent code suggestions directly within your Integrated Development Environment (IDE).
How It Works
GitHub Copilot is designed to understand natural language prompts and generate corresponding code. It can assist with writing entire functions, suggesting code snippets, and even debugging.
Example Usage
Here’s an example of how GitHub Copilot can help you write a Python function to fetch data from an API:
import requests
def fetch_data(api_url):
response = requests.get(api_url)
if response.status_code == 200:
return response.json()
else:
return None
# Prompt: Write a function to fetch user data from an API
# GitHub Copilot suggestion:
def fetch_user_data(api_url, user_id):
response = requests.get(f"{api_url}/users/{user_id}")
if response.status_code == 200:
return response.json()
else:
return None
Benefits
- Speed: Accelerates coding by providing quick suggestions.
- Accuracy: Reduces the likelihood of syntax errors.
- Learning: Helps developers learn new programming patterns and best practices.
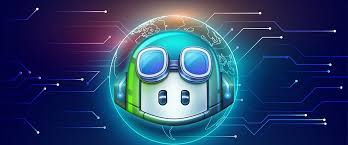
2. TabNine
TabNine is an AI-powered code completion tool that supports multiple programming languages and IDEs. It uses deep learning models to predict and suggest code snippets, improving coding speed and efficiency.
How It Works
TabNine’s deep learning model is trained on a vast amount of open-source code. It provides contextual code completions based on the code you’re writing.
Example Usage
Imagine you’re writing a JavaScript function to filter an array of numbers. TabNine can suggest the next lines of code based on your current context:
// Prompt: Write a function to filter out even numbers from an array
function filterEvenNumbers(arr) {
return arr.filter(num => num % 2 !== 0);
}
// TabNine suggestion:
function filterEvenNumbers(arr) {
return arr.filter(num => num % 2 !== 0);
}
Benefits
- Multi-language support: Works with various programming languages.
- Customization: Allows customization to better fit individual coding styles.
- Integration: Easily integrates with popular IDEs like VS Code, IntelliJ, and Sublime Text.
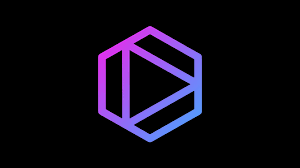
3. DeepCode
DeepCode is an AI-based static code analysis tool that detects bugs, code smells, and security vulnerabilities. It leverages machine learning models trained on billions of lines of code to provide intelligent code reviews.
How It Works
DeepCode scans your codebase and provides real-time feedback on potential issues, suggesting improvements and fixes.
Example Usage
Here’s an example of how DeepCode might identify and suggest fixing a security vulnerability in a Node.js application:
const express = require('express');
const app = express();
// Vulnerable code: No input validation
app.get('/search', (req, res) => {
const query = req.query.q;
// Perform search with query
res.send(`Search results for: ${query}`);
});
// DeepCode suggestion: Add input validation to prevent injection attacks
app.get('/search', (req, res) => {
const query = req.query.q;
if (typeof query !== 'string' || query.length > 100) {
return res.status(400).send('Invalid query');
}
// Perform search with validated query
res.send(`Search results for: ${query}`);
});
Benefits
- Security: Enhances code security by identifying vulnerabilities.
- Quality: Improves code quality by detecting bugs and code smells.
- Efficiency: Saves time by automating the code review process.
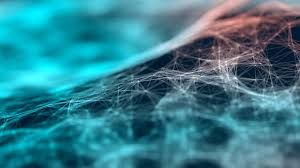
4. Kite
Kite is an AI-powered coding assistant that provides intelligent code completions and documentation. It uses machine learning models to suggest relevant code snippets and documentation as you type.
How It Works
Kite runs locally on your machine and analyzes your code to provide real-time suggestions and documentation.
Example Usage
Suppose you’re writing a Python function to manipulate strings. Kite can suggest useful string methods and provide inline documentation:
# Prompt: Write a function to reverse a string
def reverse_string(s):
return s[::-1]
# Kite suggestion and documentation:
def reverse_string(s):
"""
Reverses a given string.
Parameters:
s (str): The string to reverse.
Returns:
str: The reversed string.
"""
return s[::-1]
Benefits
- Documentation: Provides instant access to documentation, enhancing learning.
- Speed: Accelerates coding with smart completions.
- Local Processing: Runs locally, ensuring privacy and speed.

5. Codota
Codota is an AI-powered code completion tool that supports Java and Kotlin. It uses machine learning to suggest relevant code snippets and patterns based on your current context.
How It Works
Codota’s AI models are trained on large codebases, enabling it to provide contextually relevant code suggestions.
Example Usage
Let’s say you’re writing a Java function to sort a list of integers. Codota can suggest the appropriate sorting method:
import java.util.List;
import java.util.Collections;
// Prompt: Write a function to sort a list of integers
public void sortIntegers(List<Integer> numbers) {
Collections.sort(numbers);
}
// Codota suggestion:
import java.util.List;
import java.util.Collections;
public void sortIntegers(List<Integer> numbers) {
Collections.sort(numbers);
}
Benefits
- Language Support: Optimized for Java and Kotlin.
- Contextual Suggestions: Provides suggestions based on code context.
- Productivity: Boosts productivity by reducing the need to search for code snippets.
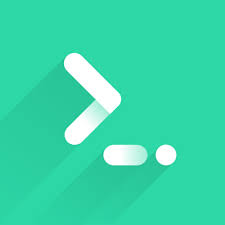
6. Intellicode
Intellicode, developed by Microsoft, is an AI-powered code recommendation tool integrated with Visual Studio and Visual Studio Code. It provides smart suggestions based on best practices from open-source projects.
How It Works
Intellicode analyzes your code and provides recommendations based on patterns found in high-quality open-source repositories.
Example Usage
If you’re writing a C# method to handle exceptions, Intellicode can suggest the best practices for exception handling:
// Prompt: Write a method to handle exceptions
public void HandleException()
{
try
{
// Code that may throw an exception
}
catch (Exception ex)
{
// Intellicode suggestion: Log the exception
Console.WriteLine(ex.Message);
}
}
Benefits
- Best Practices: Encourages best practices in coding.
- Integration: Seamlessly integrates with Visual Studio products.
- Learning: Helps developers learn from high-quality open-source projects.
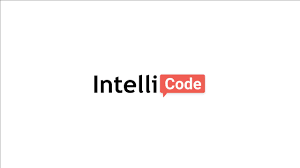
7. PyCharm with AI Assistants
PyCharm, a popular IDE for Python, integrates various AI assistants like Kite and TabNine to enhance coding productivity. These assistants provide intelligent code completions, suggestions, and documentation.
How It Works
AI assistants integrated with PyCharm analyze your code in real-time, offering relevant suggestions and documentation.
Example Usage
When writing a Python class for data processing, AI assistants can suggest methods and provide documentation:
# Prompt: Create a DataProcessor class with a method to clean data
class DataProcessor:
def clean_data(self, data):
# PyCharm with AI assistant suggestion
"""
Cleans the given data by removing null values and duplicates.
Parameters:
data (pd.DataFrame): The data to clean.
Returns:
pd.DataFrame: The cleaned data.
"""
data = data.dropna()
data = data.drop_duplicates()
return data
Benefits
- Integration: Combines the power of PyCharm with AI assistants.
- Productivity: Enhances coding speed and accuracy.
- Documentation: Provides instant access to documentation and code examples.
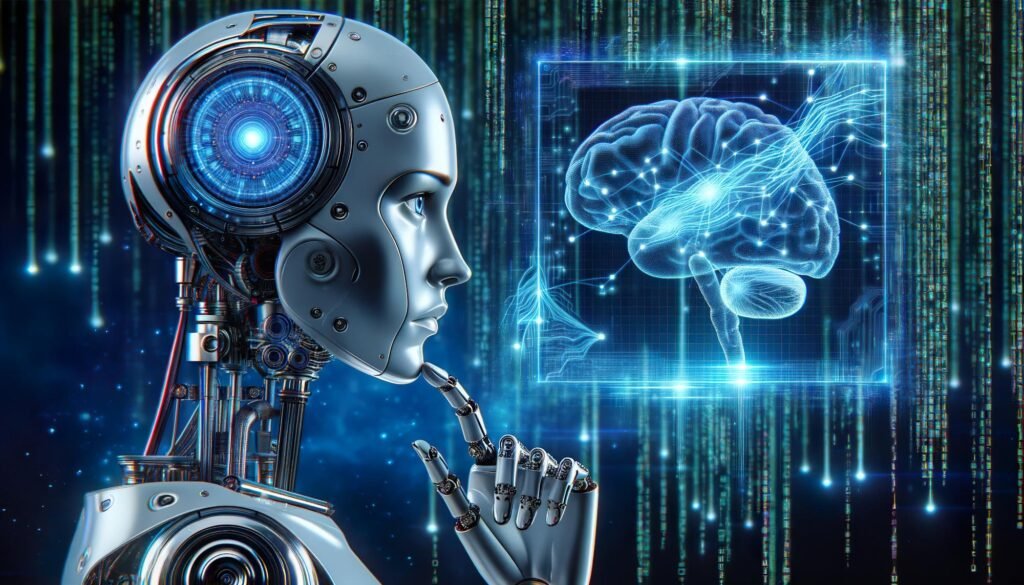
NOTE: You can also read
- SQL vs NoSQL : Understanding the Key Differences
- AI for Beginners : The complete Guide
- Python vs JavaScript: Which is Best in 2024?
- Difference Between Stack and Queue : Operations, Applications, difference, example
- C++ Arrays: Understanding and Implementing Arrays with Practical Examples
Conclusion
The integration of AI tools in software development is transforming the way developers write, debug, and manage code. Tools like GitHub Copilot, TabNine, DeepCode, Kite, Codota, Intellicode, and PyCharm with AI assistants are paving the way for a more efficient and innovative development process. By leveraging these tools, developers can not only boost their productivity but also ensure the quality and security of their code.
FAQS
What are AI tools for developers?
AI tools for developers are software applications that leverage artificial intelligence to assist developers in writing, debugging, and managing code. These tools can provide code suggestions, identify bugs and security vulnerabilities, and automate repetitive tasks to enhance productivity and code quality.
How do AI code completion tools work?
AI code completion tools, such as GitHub Copilot and TabNine, use machine learning models trained on large datasets of code. These tools analyze the context of the code being written and provide intelligent suggestions for completing code snippets, functions, or entire blocks of code.
Are AI tools for developers suitable for beginners?
Yes, AI tools can be highly beneficial for beginners. They provide real-time code suggestions, best practices, and documentation, helping new developers learn faster and write better code. Tools like GitHub Copilot and Kite are designed to assist developers at all skill levels.
2 thoughts on “7 AI Tools for Developers: Boosting Productivity and Innovation”