Difference Between Stack and Queue
Table of Contents
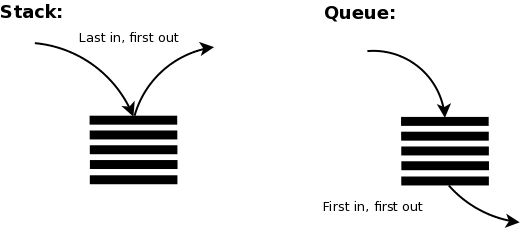
When diving into the world of computer science, understanding data structures is extremely important. Among the most basic data structures are Stack and Queue. Although they might seem almost the same at the first look, they serve different purposes and are used in different pictures/situations. In this article, we’ll explore the differences between Stack and Queue in a friendly and friendly manner. By the end, you’ll have a solid understanding of these extremely important data structures.
Introduction to Data Structures
Data structures are ways of organizing and storing data so that they can be accessed and changed (in a way that produces a lot with very little waste). Think of them as the foundation of your house. Without a solid foundation, your house won’t stand strong. (in almost the same way), without a good understanding of data structures, your programming skills won’t reach their full potential.
Two common linear data structures are stacks and waiting lines. They both allow us to store a collection of elements, but the way they handle these elements is quite different.
What is a Stack?
A stack is a linear data structure that follows the Last In, First Out (LIFO) way of thinking/basic truth/rule. Imagine a stack of plates. You can only add or remove a plate from the top. The last plate you put on the stack is the first one you’ll take off. This is exactly how a stack works in computer science.
Basic Operations on a Stack
- Push: Adding an element to the top of the stack.
- Pop: Removing the top element from the stack.
- Peek/Top: Viewing the top element without removing it.
- IsEmpty: Checking if the stack is empty.
Example of a Stack
Consider the following stack of integers:
Top -> 5
4
3
2
1
- If we push(6), the stack becomes:
Top -> 6
5
4
3
2
1
- If we pop(), the stack reverts to:
Top -> 5
4
3
2
1
Applications of Stacks
Stacks are used in various applications, including:
- Function Call Management: Keeping track of function calls and local variables.
- Undo Mechanisms: Implementing undo features in applications like text editors.
- Expression Evaluation: Evaluating arithmetic expressions and parsing syntax trees.

What is a Queue?
A queue is a linear data structure that follows the First In, First Out (FIFO) way of thinking/basic truth/rule. Imagine a line of people waiting to buy tickets. The first person in line is the first one to get the ticket. This is how a waiting line works.
Basic Operations on a Queue
- Enqueue: Adding an element to the end of the queue.
- Dequeue: Removing the front element from the queue.
- Front: Viewing the front element without removing it.
- IsEmpty: Checking if the queue is empty.
Example of a Queue
Consider the following queue of integers:
Front -> 1
2
3
4
5 <- Rear
- If we enqueue(6), the queue becomes:
Front -> 1
2
3
4
5
6 <- Rear
- If we dequeue(), the queue reverts to:
Front -> 2
3
4
5
6 <- Rear
Applications of Queues
Queues are used in various applications, including:
- Scheduling: Managing tasks in operating systems and job scheduling.
- Data Streaming: Handling data streams and buffering.
- Breadth-First Search (BFS): Traversing graphs and trees.
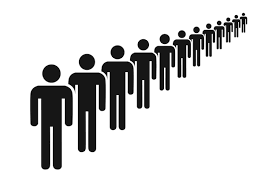
Key Differences Between Stack and Queue
Now that we have a basic understanding of stack and queues, let’s delve into the key differences between them:
- Order of Operations:
- Stack: Follows LIFO (Last In, First Out). The most recently added element is removed first.
- Queue: Follows FIFO (First In, First Out). The earliest added element is removed first.
- Insertion and Deletion Points:
- Stack: Both insertion (push) and deletion (pop) operations occur at the top of the stack.
- Queue: Insertion (enqueue) occurs at the rear, and deletion (dequeue) occurs at the front.
- Use Cases:
- Stack: Suitable for scenarios requiring reverse order processing, such as undo mechanisms and backtracking algorithms.
- Queue: Suitable for scenarios requiring sequential processing, such as task scheduling and BFS traversal.
- Implementation:
- Stack: Can be implemented using arrays or linked lists. In an array-based implementation, the top index is incremented/decremented.
- Queue: Can also be implemented using arrays or linked lists. In an array-based implementation, two indices (front and rear) are used.
Real-World Analogies
To make the concepts even clearer, let’s use real-world analogies:
- Stack: Think of a stack of books. You can only add or remove the top book. If you want to access the bottom book, you need to remove all the books above it.
- Queue: Think of a line at a coffee shop. The first person in line is the first to get served. Newcomers join the end of the line, and service proceeds from the front.
Python Implementations
Let’s see how we can implement stack and queues in Python.
Stack Implementation
class Stack:
def __init__(self):
self.items = []
def is_empty(self):
return len(self.items) == 0
def push(self, item):
self.items.append(item)
def pop(self):
if not self.is_empty():
return self.items.pop()
return None
def peek(self):
if not self.is_empty():
return self.items[-1]
return None
def size(self):
return len(self.items)
# Usage
stack = Stack()
stack.push(1)
stack.push(2)
stack.push(3)
print(stack.pop()) # Output: 3
print(stack.peek()) # Output: 2
Queue Implementation
class Queue:
def __init__(self):
self.items = []
def is_empty(self):
return len(self.items) == 0
def enqueue(self, item):
self.items.append(item)
def dequeue(self):
if not self.is_empty():
return self.items.pop(0)
return None
def front(self):
if not self.is_empty():
return self.items[0]
return None
def size(self):
return len(self.items)
# Usage
queue = Queue()
queue.enqueue(1)
queue.enqueue(2)
queue.enqueue(3)
print(queue.dequeue()) # Output: 1
print(queue.front()) # Output: 2
NOTE: You can also read
- C++ Arrays: Understanding and Implementing Arrays with Practical Examples
- Merge Sort Algorithm and Its Implementations
Conclusion
Understanding the differences between stack and queue is basic for any computer science student or professional. While both are linear data structures, their beliefs about right and wrong of operation and use cases differ significantly. Stacks are ideal for pictures/situations needing/ordering LIFO processing, whereas waiting lines are perfect for FIFO operations. By mastering these data structures, you’ll be better prepared to tackle a wide range of programming challenges.
FAQS
What is the main difference between a stack and a queue?
The main difference is in the order of operations:
Stack: Follows the Last In, First Out (LIFO) principle. The last element added is the first one to be removed.
Queue: Follows the First In, First Out (FIFO) principle. The first element added is the first one to be removed.
How do you implement a stack in Python?
A stack can be implemented in Python using a list. Key operations include:
Push: append()
method to add elements to the top.
Pop: pop()
method to remove elements from the top.
How do you implement a queue in Python
A queue can be implemented in Python using a list. Key operations include:
Enqueue: append()
method to add elements to the rear.
Dequeue: pop(0)
method to remove elements from the front.
4 thoughts on “Understanding the Difference Between Stack and Queue”